MaterialFrame MAUI: blur effect, acrylic brush, dark theme...
Add blur effect to your views on .net MAUI. But also supports Dark and Light theming, and a nice acrylic effect.
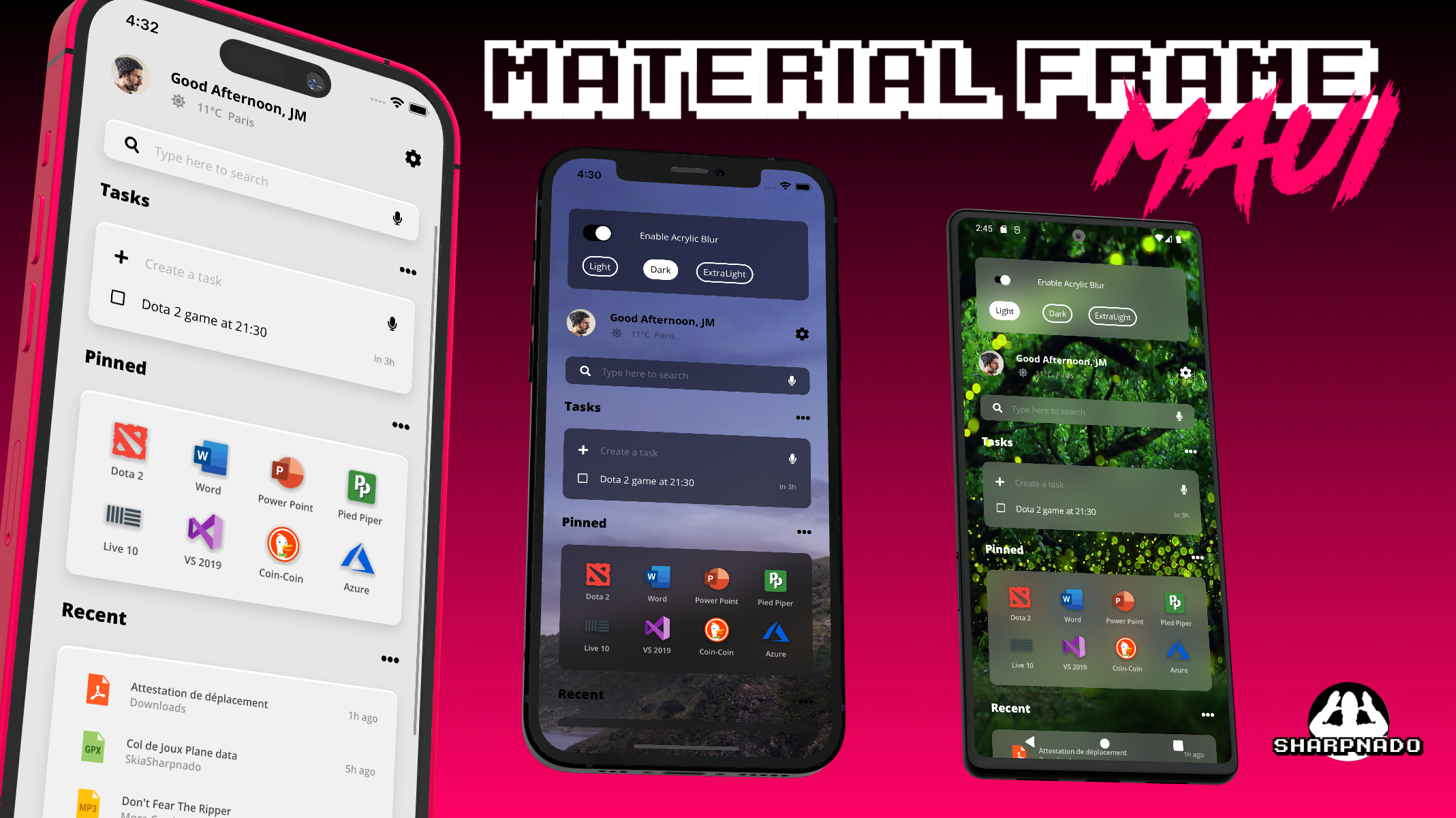
MAUI | Xamarin.Forms |
---|---|
✅ Android | ✅ Android |
✅ iOS | ✅ iOS |
❓ macOS | ✅ macOS |
✅ WinUI | ✅ UWP |
Initialization
- In
MauiProgram.cs
:
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.UseSharpnadoMaterialFrame(loggerEnable: false)
...
}
Description
After some real struggle with the MAUI early versions, the Sharpnado's MaterialFrame is finally ready for .net 7 MAUI!
It means it will support all maui apps starting from .net 7.
For those who don't know the Material Frame, it brings you blur effect on all Maui platforms. Classic material design light and dark mode with material elevations. But also a distinguished "Acrylic" mode who gives a dandy glossy render to your pale frames.

All of those modes are easily accessible from one simple property: MaterialTheme
:
public enum Theme
{
Light = 0,
Dark,
Acrylic,
AcrylicBlur,
}
Light
In light theme, you can set the LightThemeBackgroundColor
and control the Elevation
.

Dark
In dark theme, you can only control the Elevation
, more elevation equals more light on the black frame (see below).

Acrylic
In Acrylic theme, you can still set the LightThemeBackgroundColor
, also a Color
of F1F1F1
is advised to have a good Acrylic
effect.

AcrylicBlur
In AcrylicBlur theme, LightThemeBackgroundColor
and Elevation
properties are discarded.
You can set the BlurStyle
property for both Android
and iOS
.
REMARK: On Android
go easy on the blur: it's a resource intensive operation. For each blurred frame, it will go trough all the view hierarchy from the root view to the target view.
Light

ExtraLight

Dark

LightThemeBackgroundColor
The background color in Light
and Acrylic
themes. In Dark
theme, this value is ignored because the background color depends on the Elevation
. In AcrylicBlur
, the value is discarded cause iOS
doesn't allow you to control the overlay color. Note that setting the BackgroundColor
property has no effect with the MaterialFrame
.
AcrylicGlowColor
You can change the "glow" of a MaterialFrame
with an acrylic theme (the thin top white glow). Default is white. This property is ignored is the theme is not set to Acrylic.
Elevation
This property semantic changes according to the theme currently set:
Light Theme
Cast a shadow according to Google's Material elevation specs.
Dark Theme
Change the frame's background color according to Google's dark mode specs:

Examples of styles
Acrylic style
From Sharpnado.Acrylic.Maui github repo, MaterialFrame.xaml
file:
<ResourceDictionary xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:rv="clr-namespace:Sharpnado.MaterialFrame;assembly=Sharpnado.MaterialFrame">
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="Colors.xaml" />
</ResourceDictionary.MergedDictionaries>
<Style TargetType="rv:MaterialFrame">
<Setter Property="MaterialTheme" Value="Acrylic" />
<Setter Property="Margin" Value="5, 5, 5, 10" />
<Setter Property="Padding" Value="20,15" />
<Setter Property="CornerRadius" Value="10" />
<Setter Property="LightThemeBackgroundColor" Value="{StaticResource AcrylicFrameBackgroundColor}" />
</Style>
</ResourceDictionary>
Color.xaml
file:
<ResourceDictionary xmlns="xmlns="http://schemas.microsoft.com/dotnet/2021/maui"" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml">
<Color x:Key="AcrylicSurface">#E6E6E6</Color>
<Color x:Key="AcrylicFrameBackgroundColor">#F1F1F1</Color>
<Color x:Key="AccentColor">#00E000</Color>
<Color x:Key="PrimaryColor">Black</Color>
<Color x:Key="SecondaryColor">#60000000</Color>
<Color x:Key="TernaryColor">#30000000</Color>
<Color x:Key="TextPrimaryColor">Black</Color>
<Color x:Key="TextSecondaryColor">#60000000</Color>
<Color x:Key="TextTernaryColor">#40000000</Color>
</ResourceDictionary>