Dark/Light Mode: Dynamic themes in Xamarin.Forms
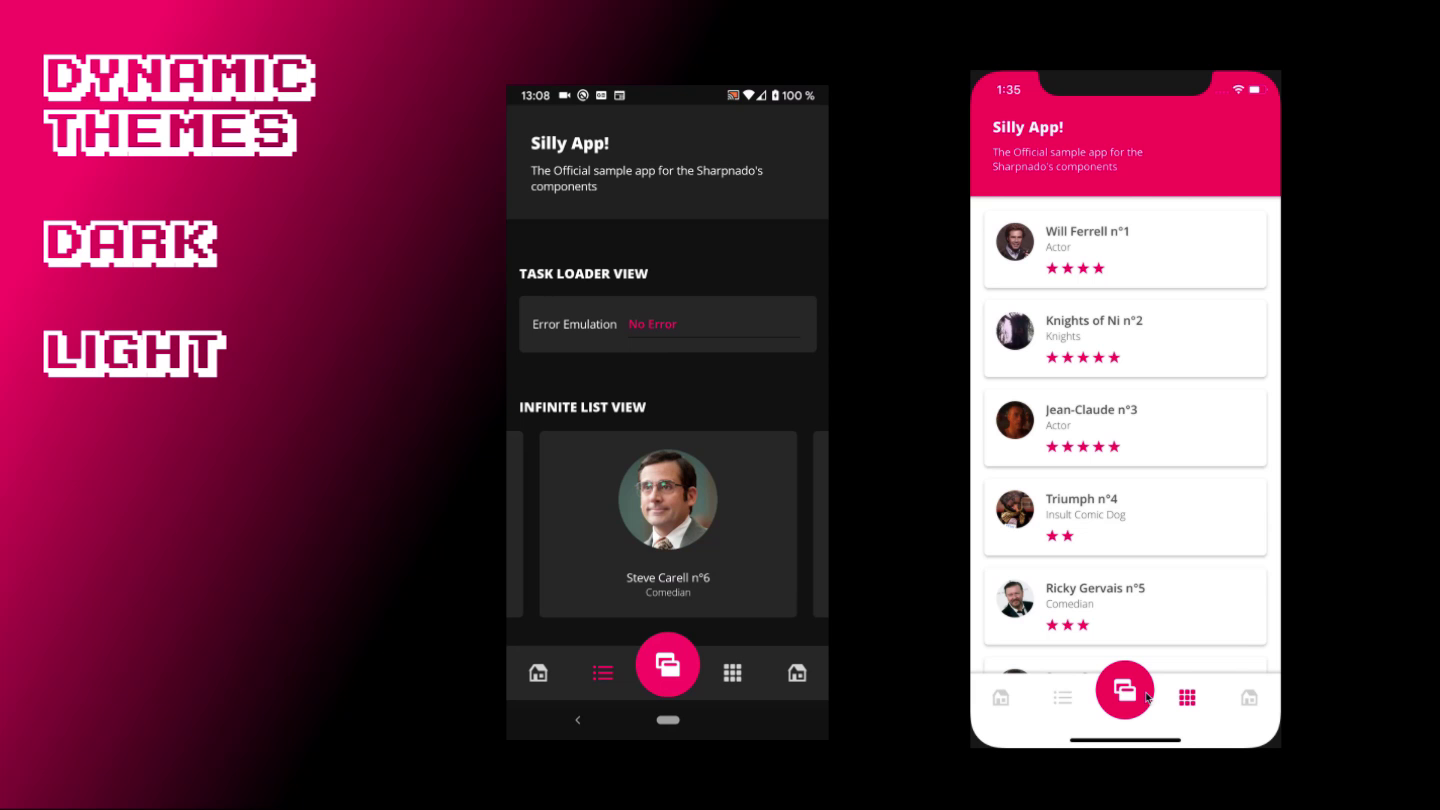
A short one today to talk about theming on our beloved Xamarin.Forms
platform.
I will use the Silly App! to showcase:
- Changing xaml properties value dynamically
- Switching between light and dark mode
- An animated transition between the themes
Android | iOS |
---|---|
![]() |
![]() |
The main impacted files by the theming are:
Changing properties dynamically
First step is to swap StaticResource
for DynamicResource
every view properties that will be impacted by your theme update.
Doing so, when you will set the DynamicBackgroundColor
from black to white for example, it will be automatically propagated to all the properties referencing this key.
Let's have a look at our App.xaml
:
<Color x:Key="DarkSurface">#121212</Color>
<Color x:Key="LightSurface">#00FF0266</Color>
...
<Style ApplyToDerivedTypes="True" TargetType="ContentPage">
<Setter Property="Padding">
<Setter.Value>
<OnPlatform x:TypeArguments="Thickness">
<On Platform="iOS">0, 20, 0, 0</On>
<On Platform="Android">0, 0, 0, 0</On>
</OnPlatform>
</Setter.Value>
</Setter>
<Setter Property="BackgroundColor" Value="{DynamicResource DynamicBackgroundColor}" />
</Style>
<Style ApplyToDerivedTypes="True" TargetType="NavigationPage">
<Setter Property="BarBackgroundColor" Value="{DynamicResource DynamicNavigationBarColor}" />
<Setter Property="BarTextColor" Value="{DynamicResource DynamicBarTextColor}" />
</Style>
We can see that DynamicResource
works also with styles...
The catch with DynamicResource
values is that you won't define it in your styles like classic StaticResource
.
For example DynamicBackgroundColor
is not defined anywhere in the xaml, you can regard them as references waiting to be assigned.
You assign them dynamically in your code like this (we'll see the SetDynamicResource
implementation right after that):
// Dark Mode
SetDynamicResource(DynamicBackgroundColor, "DarkSurface");
// Ligh Mode
SetDynamicResource(DynamicBackgroundColor, "LightSurface");
Switching between Light and Dark mode
In my Silly App! bottom bar I have a TabButton
with a toggle theme icon, clicking on it will call either SetDarkMode()
or SetLightMode()
:
namespace SillyCompany.Mobile.Practices.Presentation.Views
{
public static class ResourcesHelper
{
public const string DynamicPrimaryTextColor = nameof(DynamicPrimaryTextColor);
public const string DynamicSecondaryTextColor = nameof(DynamicSecondaryTextColor);
public const string DynamicNavigationBarColor = nameof(DynamicNavigationBarColor);
public const string DynamicBackgroundColor = nameof(DynamicBackgroundColor);
public const string DynamicBarTextColor = nameof(DynamicBarTextColor);
public const string DynamicTopShadow = nameof(DynamicTopShadow);
public const string DynamicBottomShadow = nameof(DynamicBottomShadow);
public const string DynamicHasShadow = nameof(DynamicHasShadow);
public const string Elevation4dpColor = nameof(Elevation4dpColor);
...
public static void SetDynamicResource(string targetResourceName, string sourceResourceName)
{
if (!Application.Current.Resources.TryGetValue(sourceResourceName, out var value))
{
throw new InvalidOperationException($"key {sourceResourceName} not found in the resource dictionary");
}
Application.Current.Resources[targetResourceName] = value;
}
public static void SetDynamicResource<T>(string targetResourceName, T value)
{
Application.Current.Resources[targetResourceName] = value;
}
public static void SetDarkMode()
{
MaterialFrame.ChangeGlobalTheme(MaterialFrame.Theme.Dark);
SetDynamicResource(DynamicNavigationBarColor, "DarkElevation2dp");
SetDynamicResource(DynamicBarTextColor, "TextPrimaryDarkColor");
SetDynamicResource(DynamicTopShadow, ShadowType.None);
SetDynamicResource(DynamicBottomShadow, ShadowType.None);
SetDynamicResource(DynamicHasShadow, false);
SetDynamicResource(DynamicPrimaryTextColor, "TextPrimaryDarkColor");
SetDynamicResource(DynamicSecondaryTextColor, "TextSecondaryDarkColor");
SetDynamicResource(DynamicBackgroundColor, "DarkSurface");
SetDynamicResource(Elevation4dpColor, "DarkElevation4dp");
}
public static void SetLightMode()
{
MaterialFrame.ChangeGlobalTheme(MaterialFrame.Theme.Light);
SetDynamicResource(DynamicNavigationBarColor, "Accent");
SetDynamicResource(DynamicBarTextColor, "TextPrimaryDarkColor");
SetDynamicResource(DynamicTopShadow, ShadowType.Top);
SetDynamicResource(DynamicBottomShadow, ShadowType.Bottom);
SetDynamicResource(DynamicHasShadow, true);
SetDynamicResource(DynamicPrimaryTextColor, "TextPrimaryLightColor");
SetDynamicResource(DynamicSecondaryTextColor, "TextSecondaryLightColor");
SetDynamicResource(DynamicBackgroundColor, "LightSurface");
SetDynamicResource(Elevation4dpColor, "OnSurfaceColor");
}
}
}
You can see that I am not only changing colors, but also disabling shadows in dark mode, since dark modes are flat by essence.
Example: SillyBottomTabsPage.cs
<tb:Toolbar x:Name="Toolbar"
Title="Silly App!"
BackgroundColor="{DynamicResource DynamicNavigationBarColor}"
ForegroundColor="White"
HasShadow="{DynamicResource DynamicHasShadow}"
Subtitle="The Official sample app for the Sharpnado's components" />
...
<tabs:TabHostView x:Name="TabHost"
Grid.Row="2"
BackgroundColor="{DynamicResource Elevation4dpColor}"
ShadowType="{DynamicResource DynamicTopShadow}"
TabType="Fixed"
SelectedIndex="{Binding Source={x:Reference Switcher}, Path=SelectedIndex, Mode=TwoWay}">
<tabs:TabButton x:Name="TabButton"
IsVisible="True"
ButtonBackgroundColor="{StaticResource Accent}"
ButtonCircleSize="60"
ButtonPadding="15"
IconImageSource="theme_96.png"
Scale="1.3"
TranslationY="-10"
Clicked="TabButtonOnClicked" />
...
Making the transition
And now let's see the code for our transition:
SillyBottomTabsPage.xaml.cs
private void TabButtonOnClicked(object sender, EventArgs e)
{
TaskMonitor.Create(AnimateTabButton);
}
private void ApplyTheme()
{
if (_currentTheme == Theme.Light)
{
ResourcesHelper.SetLightMode();
return;
}
ResourcesHelper.SetDarkMode();
}
private async Task AnimateTabButton()
{
double sourceScale = TabButton.Scale;
Color sourceColor = TabButton.ButtonBackgroundColor;
Color targetColor = _currentTheme == Theme.Light
? ResourcesHelper.GetResourceColor("DarkSurface")
: Color.White;
// Bounce then remove icon from button,
await TabButton.ScaleTo(3);
await TabButton.ScaleTo(sourceScale);
TabButton.IconImageSource = null;
// Ballon inflation
var bigScaleTask = TabButton.ScaleTo(30, length: 500);
// Change color to target dark/light mode
var colorChangeTask = TabButton.ColorTo(
sourceColor,
targetColor,
callback: c => TabButton.ButtonBackgroundColor = c,
length: 500);
// run animation at the same time
await Task.WhenAll(bigScaleTask, colorChangeTask);
_currentTheme = _currentTheme == Theme.Light ? Theme.Dark : Theme.Light;
ApplyTheme();
// reverse inflation and color animation to accent color
var reverseBigScaleTask = TabButton.ScaleTo(sourceScale, length: 500);
var reverseColorChangeTask = TabButton.ColorTo(
targetColor,
sourceColor,
c => TabButton.ButtonBackgroundColor = c,
length: 500);
await Task.WhenAll(reverseBigScaleTask, reverseColorChangeTask);
// icon is back
TabButton.IconImageSource = "theme_96.png";
}
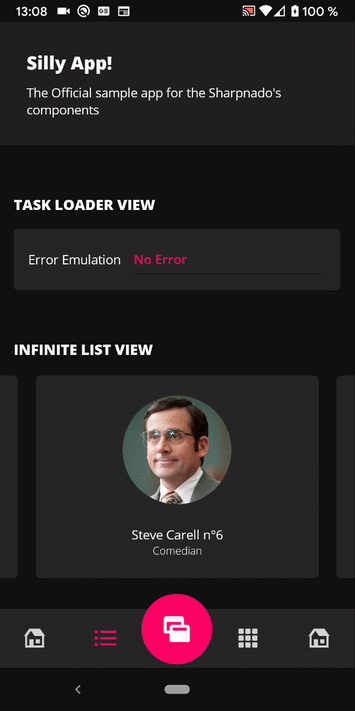
Some design considerations
In fact the hardest thing in theming is having a coherent design for light and dark mode. You want to keep your accent color since it is part of your app identity.
For light theme, I found that taking the accent color with the alpha channel to zero is working nicely as a background color.